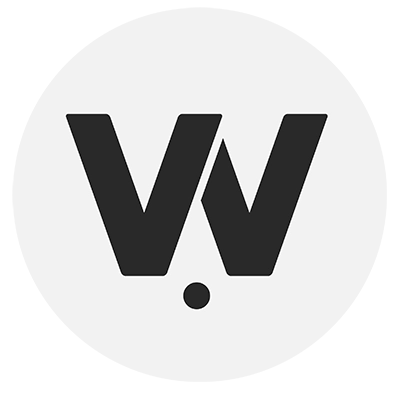
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/account",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/account" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/account",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[StoreID] => Integer
[UserName] => String
[StoreName] => String
[StoreAddress] => String
[StoreAddressNo] => String
[StorePostalCode] => String
[StoreCity] => String
[StoreVAT] => String
[StorePhone] => String
)
)
Dette API-endepunkt gør det muligt for en autoriseret bruger at hente en liste over alle medarbejdere. Det kræver, at brugeren er autentificeret.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/employees",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/employees" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/employees",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
Denne endpoint tillader sletning af en specifik ressource identificeret ved dens unikke ID. Når anmodningen udføres korrekt, fjernes ressourcen permanent fra systemet.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/clients/{id}",
CURLOPT_CUSTOMREQUEST => "DEL",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request DEL "https://api.wiggo.dk/v2/clients/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/clients/{id}",
"method": "DEL",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[Success] => String
)
)
Denne endpoint returnerer en liste over alle registrerede klienter i systemet. Anmodningen kræver ingen specifikke parametre og returnerer den komplette klientliste i JSON-format.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/clients/?limit={100}&from={YY-MM-DD}&to={YY-MM-DD}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/clients/?limit={100}&from={YY-MM-DD}&to={YY-MM-DD}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/clients/?limit={100}&from={YY-MM-DD}&to={YY-MM-DD}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ClientID] => Integer
[FirmName] => String
[Firstname] => String
[Lastname] => String
[PhoneCode] => String
[Phone] => String
[Email] => String
[Date] => DateTime()
[Saldo] => String
)
)
Hent specifik Client baseret på ClientID. Kan multipliceres ved kommaseperation.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/clients/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/clients/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/clients/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ClientID] => Integer
[FirmName] => String
[Firstname] => String
[Lastname] => String
[PhoneCode] => String
[Phone] => String
[Email] => String
[Date] => String
[Saldo] => String
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/clients/?FirstName={str}&LastName={str}&Email={str}&PhoneCode={int}&Phone={int}",
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request POST "https://api.wiggo.dk/v2/clients/?FirstName={str}&LastName={str}&Email={str}&PhoneCode={int}&Phone={int}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/clients/?FirstName={str}&LastName={str}&Email={str}&PhoneCode={int}&Phone={int}",
"method": "POST",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ClientID] => Integer
)
)
Denne endpoint giver mulighed for at opdatere oplysninger om eksisterende klienter i systemet.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/clients/{id}",
CURLOPT_CUSTOMREQUEST => "PUT",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request PUT "https://api.wiggo.dk/v2/clients/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/clients/{id}",
"method": "PUT",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
Denne endpoint tillader sletning af en specifik ressource identificeret ved dens unikke ID. Når anmodningen udføres korrekt, fjernes ressourcen permanent fra systemet.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/products/{id}",
CURLOPT_CUSTOMREQUEST => "DEL",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request DEL "https://api.wiggo.dk/v2/products/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/products/{id}",
"method": "DEL",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/productgroups",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/productgroups" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/productgroups",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/productgroups/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/productgroups/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/productgroups/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/products/?limit={100}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/products/?limit={100}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/products/?limit={100}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ProductID] => Integer
[HideFromWeb] => Integer
[Title] => String
[Url] => String
[Description] => String
[Price] => Integer
[OfferPrice] => Integer
[Date] => DateTime()
[GroupTitle] => String
[GroupID] => Integer
[Brand] => String
[BrandID] => Integer
[BrandLink] => String
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/products/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/products/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/products/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/invoices/?from={YY-MM-DD}&to={YY-MM-DD}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/invoices/?from={YY-MM-DD}&to={YY-MM-DD}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/invoices/?from={YY-MM-DD}&to={YY-MM-DD}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[Customer] => Array()
[InvoiceID] => Integer
[InvoiceNo] => Integer
[Amount] => Integer
[Tax] => String
[InvoiceDate] => DateTime()
[DueDate] => DateTime()
[OrderID] => Integer
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/invoices/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/invoices/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/invoices/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[Customer] => Array()
[InvoiceLines] => Array()
[InvoiceID] => Integer
[InvoiceNo] => Integer
[Amount] => Integer
[Tax] => Integer
[InvoiceDate] => DateTime()
[DueDate] => DateTime()
[OrderID] => Integer
)
)
Alle ordre listet, med mulighed for filtrering
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/orders",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/orders" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/orders",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[OrderID] => Integer
[OrderNo] => Integer
[InvoiceNo] => Integer
[Terminal] => Integer
[Date] => DateTime()
[Customer] => Array()
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/orders/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/orders/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/orders/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[OrderID] => Integer
[OrderNo] => Integer
[InvoiceNo] => Integer
[Terminal] => Integer
[Date] => DateTime()
[Customer] => Array()
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/orders",
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request POST "https://api.wiggo.dk/v2/orders" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/orders",
"method": "POST",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/stocklocations",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/stocklocations" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/stocklocations",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/stockreports",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/stockreports" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/stockreports",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/articles",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/articles" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/articles",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ArticleID] => Integer
[Page] => String
[Title] => String
[TitleURL] => String
[Created] => String
[Tags] => String
[Image] => String
[Image_full] => String
)
)
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/articles/{id}",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/articles/{id}" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/articles/{id}",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[ArticleID] => Integer
[Page] => String
[Title] => String
[TitleURL] => String
[Created] => DateTime()
[Tags] => String
[Image] => String
[Image_full] => String
)
)
Alle sider oprettet i backend.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.wiggo.dk/v2/pages",
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_USERAGENT => "wiggo",
CURLOPT_HTTPHEADER => array(
"AppSecretToken: {key}"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
curl --location --request GET "https://api.wiggo.dk/v2/pages" \
--header "AppSecretToken: {key}" \
--data ""
}
var settings = {
"url": "https://api.wiggo.dk/v2/pages",
"method": "GET",
"timeout": 0,
"headers": {
"AppSecretToken": "{key}"
},
};
Array
(
[0] => stdClass Object
(
[PagesID] => Integer
[Type] => String
[Template] => String
[Logo] => String
[MainTitle] => String
[ContactMail] => String
[Menubar] => Array()
[Title] => String
[Url] => String
[Description] => String
[Meta] => String
[Rotations] => Array()
[Content] => String
[Information] => Array()
)
)